String Literals
- 문자열 리터럴은 큰따옴표로 둘러싸인 문자열을 말하며 아래의 예시와 같습니다.
let someString = "Some string literal value"
- 다중행 문자열 리터럴은 세 개의 큰따옴표로 둘러싸인 문자열을 말하며 아래의 예시와 같습니다.
let quotation = """
The White Rabbit put on his spectacles. "Where shall I begin,
please your Majesty?" he asked.
"Begin at the beginning," the King said gravely, "and go on
till you come to the end; then stop."
"""
- 다중행 문자열 리터럴은 그 시작과 끝 따옴표 사이의 모든 라인을 포함합니다. 문자열은 첫 번째 줄에서 시작되며, 첫 번째 줄에서 시작됩니다. (""")로 시작하며 (""")로 끝나므로 부호가 없을 시 종료되지 않습니다. 또한 문자열 리터럴 내부에서 줄 바꿈을 사용할 때 그 줄 바꿈이 문자열 값에 포함되지 않게 하려면 \를 사용합니다.
let softWrappedQuotation = """
The White Rabbit put on his spectacles. "Where shall I begin, \
please your Majesty?" he asked.
"Begin at the beginning," the King said gravely, "and go on \
till you come to the end; then stop."
"""
- 시작 따옴표 바로 뒤의 공백은 Swift에게 다른 모든 행보다 먼저 무시해야 할 공백을 알려줍니다 . (Space Ignored)
그러나, 끝 따옴표 이전의 것 외에 행의 시작 부분에 공백을 쓰면, 그 공백이 포함됩니다. (Appears in String)
Special Characters in String Literals
- \0 (이중 캐릭터),
\\ (백슬래시),
\t (필수 탭),
\n (라인 피드),
\r (반환),
\" (더블 인용 부호) 및 \' (싱글 따옴표)
임의 유니코드 스칼라 값(으)으로 기록됩니다. \u {n} ( n = 1~8자리 16진수) 등의 특수문자가 존재하며 아래는 예시입니다.
let wiseWords = "\"Imagination is more important than knowledge\" - Einstein"
// "Imagination is more important than knowledge" - Einstein
let dollarSign = "\u{24}" // $, Unicode scalar U+0024
let blackHeart = "\u{2665}" // ♥, Unicode scalar U+2665
let sparklingHeart = "\u{1F496}" // 💖, Unicode scalar U+1F496
- 다중 문자열 안에서 이스케이프를 포함하는 형태는 아래의 예시와 같습니다.
let threeDoubleQuotationMarks = """
Escaping the first quotation mark \"""
Escaping all three quotation marks \"\"\"
"""
Initializing an Empty String
- 아래의 예시와 같이 초기화 할 수 있습니다.
var emptyString = "" // empty string literal
var anotherEmptyString = String() // initializer syntax
// these two strings are both empty, and are equivalent to each other
- 또한 빈 값인지는 IsEmpty 를 사용하여 확인할 수 있습니다.
if emptyString.isEmpty {
print("Nothing to see here")
}
// Prints "Nothing to see here"
String Mutability
var variableString = "Horse"
variableString += " and carriage"
// variableString is now "Horse and carriage"
let constantString = "Highlander"
constantString += " and another Highlander"
// this reports a compile-time error - a constant string cannot be modified
Working with Characters
- 문자열에 접근할 수 있습니다. 아래는 이해를 돕기 위한 예시입니다.
for character in "Dog!🐶" {
print(character)
}
// D
// o
// g
// !
// 🐶
- Character 타입의 배열을 형변환하여 출력하는 예시입니다.
let catCharacters: [Character] = ["C", "a", "t", "!", "🐱"]
let catString = String(catCharacters)
print(catString)
// Prints "Cat!🐱"
Concatenating Strings and Characters
- String 값은 추가 연산자와 함께 연결할 수 있습니다.
let string1 = "hello"
let string2 = " there"
var welcome = string1 + string2
// welcome now equals "hello there"
- (+=) 사용
var instruction = "look over"
instruction += string2
// instruction now equals "look over there"
- append() 사용
let exclamationMark: Character = "!"
welcome.append(exclamationMark)
// welcome now equals "hello there!"
- 다중행 문자열 리터럴을 사용시 문자열의 모든 행이 마지막 줄을 포함하여 줄 바꿈으로 끝나기를 원할 경우 아래와 같은 예시를 사용할 수 있습니다.
let badStart = """
one
two
"""
let end = """
three
"""
print(badStart + end)
// Prints two lines:
// one
// twothree
let goodStart = """
one
two
"""
print(goodStart + end)
// Prints three lines:
// one
// two
// three
String Interpolation
- 문자열 리터럴에 삽입하는 각 항목은 백슬래시() 로 앞에 붙은 괄호 쌍으로 싸여 있습니다.
let multiplier = 3
let message = "\(multiplier) times 2.5 is \(Double(multiplier) * 2.5)"
// message is "3 times 2.5 is 7.5"
- 확장 문자열 구분 기호를 사용하여 문자열 보간으로 간주되는 문자를 포함하는 문자열을 만들 수 있습니다. 예를 들면 다음과 같습니다.
print(#"Write an interpolated string in Swift using \(multiplier)."#)
// Prints "Write an interpolated string in Swift using \(multiplier)."
- 확장 구분 기호를 사용하는 문자열 내에서 문자열 보간법을 사용하려면 백 슬래시 후 숫자 기호의 수를 문자열의 처음과 끝에 있는 숫자 기호의 수와 일치시켜서 사용할 수 있습니다. 예를 들면 다음과 같습니다.
print(#"6 times 7 is \#(6 * 7)."#)
// Prints "6 times 7 is 42."
Unicode
- 유니코드는 서로 다른 쓰기 시스템에서 텍스트를 인코딩, 표현, 처리하기 위한 국제 표준입니다. 표준화된 형태로 어떤 언어에서든 거의 모든 문자를 표현할 수 있고, 텍스트 파일이나 웹 페이지와 같은 외부 소스에서 읽고 쓸 수 있습니다. 스위프트에서는 String 그리고 Character 형식은 완전한 유니코드 호환을 지원합니다.
let precomposed: Character = "\u{D55C}" // 한
let decomposed: Character = "\u{1112}\u{1161}\u{11AB}" // ᄒ, ᅡ, ᆫ
// precomposed is 한, decomposed is 한
Counting Characters
- count를 사용하여 문자열의 숫자를 카운트 할 수 있으며 아래의 예시와 같습니다.
let unusualMenagerie = "Koala 🐨, Snail 🐌, Penguin 🐧, Dromedary 🐪"
print("unusualMenagerie has \(unusualMenagerie.count) characters")
// Prints "unusualMenagerie has 40 characters"
var word = "cafe"
print("the number of characters in \(word) is \(word.count)")
// Prints "the number of characters in cafe is 4"
word += "\u{301}" // COMBINING ACUTE ACCENT, U+0301
print("the number of characters in \(word) is \(word.count)")
// Prints "the number of characters in café is 4"
Accessing and Modifying a String
- startIndex는 문자열의 첫번째 endIndex는 문자열의 마지막에 접근합니다.
let greeting = "Guten Tag!"
greeting[greeting.startIndex]
// G
greeting[greeting.index(before: greeting.endIndex)]
// !
greeting[greeting.index(after: greeting.startIndex)]
// u
let index = greeting.index(greeting.startIndex, offsetBy: 7)
greeting[index]
// a
- 문자열의 범위 또는 범위를 벗어나는 인덱스에 액세스 하려고 시도하는 중 Character 문자열 범위를 벗어나는 인덱스에서 런타임 오류를 트리거합니다.
greeting[greeting.endIndex] // Error
greeting.index(after: greeting.endIndex) // Error
- 문자열에서 개별 문자의 모든 인덱스에 액세스 할 수 있는 속성의 예시입니다.
for index in greeting.indices {
print("\(greeting[index]) ", terminator: "")
}
// Prints "G u t e n T a g ! "
Inserting and Removing
- 문자열에 대한 삽입에 대한 예시입니다.
var welcome = "hello"
welcome.insert("!", at: welcome.endIndex)
// welcome now equals "hello!"
welcome.insert(contentsOf: " there", at: welcome.index(before: welcome.endIndex))
// welcome now equals "hello there!"
- 문자열에 대한 삭제에 대한 예시입니다.
welcome.remove(at: welcome.index(before: welcome.endIndex))
// welcome now equals "hello there"
let range = welcome.index(welcome.endIndex, offsetBy: -6)..<welcome.endIndex
welcome.removeSubrange(range)
// welcome now equals "hello"
Substrings
let greeting = "Hello, world!"
let index = greeting.firstIndex(of: ",") ?? greeting.endIndex
let beginning = greeting[..<index]
// beginning is "Hello"
// Convert the result to a String for long-term storage.
let newString = String(beginning)
- 문자열과 마찬가지로 각 하위 문자열에는 하위 문자열을 구성하는 문자가 저장되는 메모리 영역이 있습니다. 문자열과 하위 문자열의 차이는 성능 최적화로서 하위 문자열은 원래 문자열을 저장하는 데 사용되는 메모리의 일부 또는 다른 하위 문자열을 저장하는 데 사용되는 메모리의 일부를 재사용할 수 있습니다.
이러한 성능 최적화는 문자열이나 하위 문자열을 수정할 때까지 메모리 복사 성능 비용을 지불하지 않아도 된다는 것을 의미합니다. 서브 문자열은 장기 저장에 적합하지 않고 원본 문자열의 저장소를 재사용하기 때문에, 원본 문자열 전체를 사용 중인 한 메모리에 보관해야 합니다.
위의 예에서, greeting 은 문자열이며, 이는 문자열을 구성하는 문자가 저장되는 메모리 영역을 가지고 있다는 것을 의미합니다. 아래의 그림을 보면 이해가 쉽습니다.
String and Character Equality
let quotation = "We're a lot alike, you and I."
let sameQuotation = "We're a lot alike, you and I."
if quotation == sameQuotation {
print("These two strings are considered equal")
}
// Prints "These two strings are considered equal"
Prefix and Suffix Equality
let romeoAndJuliet = [
"Act 1 Scene 1: Verona, A public place",
"Act 1 Scene 2: Capulet's mansion",
"Act 1 Scene 3: A room in Capulet's mansion",
"Act 1 Scene 4: A street outside Capulet's mansion",
"Act 1 Scene 5: The Great Hall in Capulet's mansion",
"Act 2 Scene 1: Outside Capulet's mansion",
"Act 2 Scene 2: Capulet's orchard",
"Act 2 Scene 3: Outside Friar Lawrence's cell",
"Act 2 Scene 4: A street in Verona",
"Act 2 Scene 5: Capulet's mansion",
"Act 2 Scene 6: Friar Lawrence's cell"
]
- 위의 배열 값 중 앞의 문자열을 hasPrefix를 사용하여 아래와 같이 다룰 수 있습니다.
var act1SceneCount = 0
for scene in romeoAndJuliet {
if scene.hasPrefix("Act 1 ") {
act1SceneCount += 1
}
}
print("There are \(act1SceneCount) scenes in Act 1")
// Prints "There are 5 scenes in Act 1"
- 위의 배열 값중 뒤의 문자열을 hasSuffix를 사용하여 아래와 같이 다룰 수 있습니다.
var mansionCount = 0
var cellCount = 0
for scene in romeoAndJuliet {
if scene.hasSuffix("Capulet's mansion") {
mansionCount += 1
} else if scene.hasSuffix("Friar Lawrence's cell") {
cellCount += 1
}
}
print("\(mansionCount) mansion scenes; \(cellCount) cell scenes")
// Prints "6 mansion scenes; 2 cell scenes"
Unicode Representations of Strings
let dogString = "Dog‼🐶"
- UTF-8 은 아래와 같이 표현할 수 있습니다.
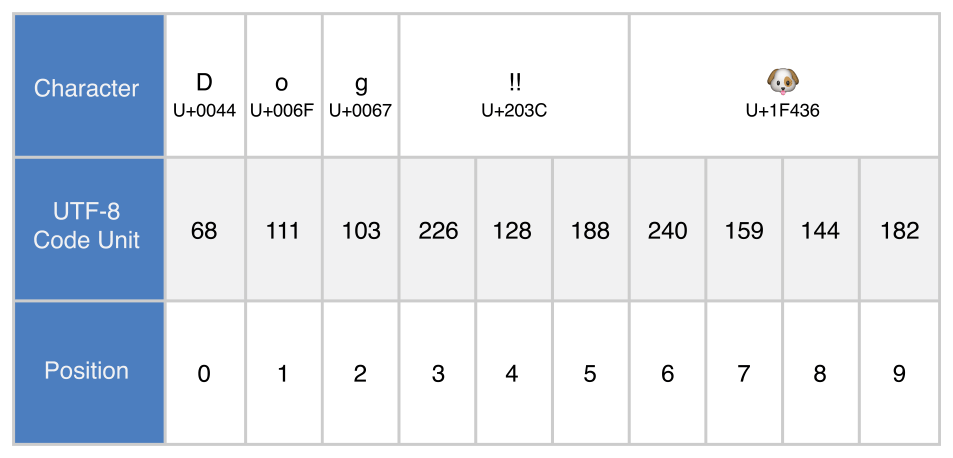
for codeUnit in dogString.utf8 {
print("\(codeUnit) ", terminator: "")
}
print("")
// Prints "68 111 103 226 128 188 240 159 144 182 "
- UTF-16 은 아래와 같이 표현할 수 있습니다.
for codeUnit in dogString.utf16 {
print("\(codeUnit) ", terminator: "")
}
print("")
// Prints "68 111 103 8252 55357 56374 "
- 유니코드 스칼라에 아래와 같이 접근할 수 있습니다.
for scalar in dogString.unicodeScalars {
print("\(scalar.value) ", terminator: "")
}
print("")
// Prints "68 111 103 8252 128054 "
for scalar in dogString.unicodeScalars {
print("\(scalar) ")
}
// D
// o
// g
// ‼
// 🐶
내용은 https://docs.swift.org/swift-book/LanguageGuide/TheBasics.html를 보면서 작성하였고 원문으로 작성된 내용을 옮기다 보니 이상한 부분이 있을 수 있습니다. 자세한 내용은 위의 링크를 확인해주시기 바랍니다.
'컴퓨터 언어 > Swift' 카테고리의 다른 글
(Swift) 5. Functions (함수) (0) | 2021.12.07 |
---|---|
(Swift) 4. Control Flow (제어문) (0) | 2021.12.07 |
(Swift) 3. Collection Types (컬렉션 타입) (0) | 2021.12.06 |
(Swift) 1. Basic Operators (기본 연산자) (0) | 2021.12.05 |
(Swift) 0. The Basics (0) | 2021.12.04 |