1. For-In Loops
- 아래는 For - in 루프를 사용한 예시입니다.
<swift />
let names = ["Anna", "Alex", "Brian", "Jack"]
for name in names {
print("Hello, \(name)!")
}
// Hello, Anna!
// Hello, Alex!
// Hello, Brian!
// Hello, Jack!
- 아래는 For - in 루프를 통해 튜플 값을 가져오는 예시입니다.
<swift />
let numberOfLegs = ["spider": 8, "ant": 6, "cat": 4]
for (animalName, legCount) in numberOfLegs {
print("\(animalName)s have \(legCount) legs")
}
// cats have 4 legs
// ants have 6 legs
// spiders have 8 legs
- 아래는 For - in 루프를 통해 가져온 값을 계산한 예시입니다.
<swift />
for index in 1...5 {
print("\(index) times 5 is \(index * 5)")
}
// 1 times 5 is 5
// 2 times 5 is 10
// 3 times 5 is 15
// 4 times 5 is 20
// 5 times 5 is 25
- 아래는 For - in 루프를 통해 가져온 값이 필요하지 않아서 ( _ )를 이용하여 생략한 예시입니다.
<swift />
let base = 3
let power = 10
var answer = 1
for _ in 1...power {
answer *= base
}
print("\(base) to the power of \(power) is \(answer)")
// Prints "3 to the power of 10 is 59049"
- 아래는 Half-open range operator 를 이용한 예시입니다.
<swift />
let minutes = 60
for tickMark in 0..<minutes {
// render the tick mark each minute (60 times)
}
- 아래는 (from , to, by) 를 이용하여 특정 조건값을 설정한 예시입니다.
<swift />
let minuteInterval = 5
for tickMark in stride(from: 0, to: minutes, by: minuteInterval) {
// render the tick mark every 5 minutes (0, 5, 10, 15 ... 45, 50, 55)
}
- 아래는 (from ,thorugh, by)를 이용하여 특정 조건값을 설정한 예시입니다.
<swift />
let hours = 12
let hourInterval = 3
for tickMark in stride(from: 3, through: hours, by: hourInterval) {
// render the tick mark every 3 hours (3, 6, 9, 12)
}
2. While Loops
- While은 값이 성립하지 않을 때 까지 계속해서 반복합니다. 아래는 일반적인 While문의 형태입니다.
<swift />
while condition {
statements
}
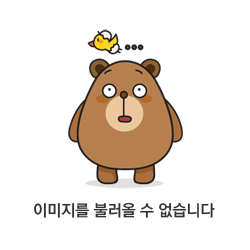
- 해당 게임의 규칙입니다.
- 보드는 25개의 정사각형을 가지고 있으며, 목표는 25번 발판에 도착하는 것입니다.
- 플레이어의 출발 광장은 왼쪽 하단의 0번 발판입니다.
- 각 회전마다 6면 주사위를 굴려 위의 점 화살표로 표시된 수평 경로를 따라 정사각형 수로 이동합니다.
- 사다리의 바닥에서 끝나면, 너는 그 사다리의 위의 발판으로 올라갑니다.
- 네 차례가 뱀의 머리에서 끝나면, 뱀의 꼬리의 발판으로 이동합니다.
- 해당 게임의 해결 방법입니다.
<swift />
let finalSquare = 25
var board = [Int](repeating: 0, count: finalSquare + 1)
//사다리
board[03] = +08;
board[06] = +11;
board[09] = +09;
board[10] = +02;
//뱀
board[14] = -10;
board[19] = -11;
board[22] = -02;
board[24] = -08;
var square = 0
var diceRoll = 0
while square < finalSquare {
// roll the dice
diceRoll += 1
if diceRoll == 7 { diceRoll = 1 }
// move by the rolled amount
square += diceRoll
if square < board.count {
// if we're still on the board, move up or down for a snake or a ladder
square += board[square]
}
}
print("Game over!")
3. Repeat While
- Repeat While은 우선 Repeat 문을 실행 후 뒤의 While문의 조건이 만족하지 않을 때까지 반복합니다. 아래는 일반적인 형태입니다.
<swift />
repeat {
statements
} while condition
- Repeat While을 사용하여 뱀과 사다리 게임을 해결한 예시입니다.
<swift />
repeat {
// move up or down for a snake or ladder
square += board[square]
// roll the dice
diceRoll += 1
if diceRoll == 7 { diceRoll = 1 }
// move by the rolled amount
square += diceRoll
} while square < finalSquare
print("Game over!")
4. If
- 물이 어는 온도인 화씨 32도 이하인지를 계산하는 예시입니다.
<swift />
var temperatureInFahrenheit = 30
if temperatureInFahrenheit <= 32 {
print("It's very cold. Consider wearing a scarf.")
}
// Prints "It's very cold. Consider wearing a scarf.
- if - else if에 대한 예시입니다.
<swift />
temperatureInFahrenheit = 90
if temperatureInFahrenheit <= 32 {
print("It's very cold. Consider wearing a scarf.")
} else if temperatureInFahrenheit >= 86 {
print("It's really warm. Don't forget to wear sunscreen.")
} else {
print("It's not that cold. Wear a t-shirt.")
}
// Prints "It's really warm. Don't forget to wear sunscreen."
5. Switch
- Switch의 기본적인 형태입니다.
<swift />
switch some value to consider {
case value 1:
respond to value 1
case value 2,
value 3:
respond to value 2 or 3
default:
otherwise, do something else
}
- Switch에서 특정 Character 값을 확인한 예시입니다.
<swift />
let someCharacter: Character = "z"
switch someCharacter {
case "a":
print("The first letter of the alphabet")
case "z":
print("The last letter of the alphabet")
default:
print("Some other character")
}
// Prints "The last letter of the alphabet"
- Swift의 Switch에서는 아래와 같이 사용 시 에러를 발생시킵니다.
<swift />
let anotherCharacter: Character = "a"
switch anotherCharacter {
case "a": // Invalid, the case has an empty body
case "A":
print("The letter A")
default:
print("Not the letter A")
}
// This will report a compile-time error.
- 이점을 해결하기 위해 아래와 같이 사용합니다.
<swift />
let anotherCharacter: Character = "a"
switch anotherCharacter {
case "a", "A":
print("The letter A")
default:
print("Not the letter A")
}
// Prints "The letter A"
6. Interval Matching
- 아래의 예시는 숫자를 입력받아 많은 정도를 반환하는 예시입니다.
<swift />
let approximateCount = 62
let countedThings = "moons orbiting Saturn"
let naturalCount: String
switch approximateCount {
case 0:
naturalCount = "no"
case 1..<5:
naturalCount = "a few"
case 5..<12:
naturalCount = "several"
case 12..<100:
naturalCount = "dozens of"
case 100..<1000:
naturalCount = "hundreds of"
default:
naturalCount = "many"
}
print("There are \(naturalCount) \(countedThings).")
// Prints "There are dozens of moons orbiting Saturn."
7. Tuples
- Switch로 튜플의 값을 검정하는 예시입니다.
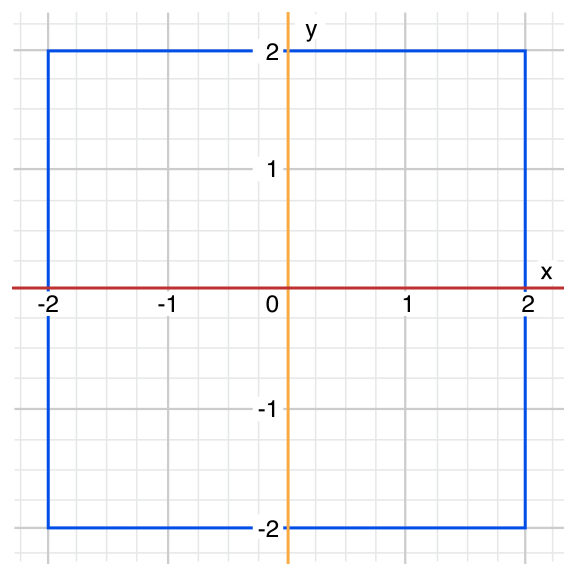
<swift />
let somePoint = (1, 1)
switch somePoint {
case (0, 0):
print("\(somePoint) is at the origin")
case (_, 0):
print("\(somePoint) is on the x-axis")
case (0, _):
print("\(somePoint) is on the y-axis")
case (-2...2, -2...2):
print("\(somePoint) is inside the box")
default:
print("\(somePoint) is outside of the box")
}
// Prints "(1, 1) is inside the box"
8. Value Bindings

<swift />
let anotherPoint = (2, 0)
switch anotherPoint {
case (let x, 0):
print("on the x-axis with an x value of \(x)")
case (0, let y):
print("on the y-axis with a y value of \(y)")
case let (x, y):
print("somewhere else at (\(x), \(y))")
}
// Prints "on the x-axis with an x value of 2"
9. Where
- Switch문에 where를 붙여 조건을 추가할 수 있습니다.
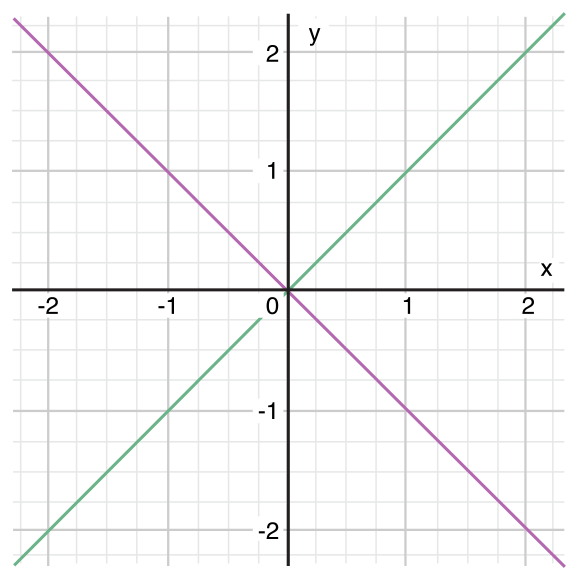
<swift />
let yetAnotherPoint = (1, -1)
switch yetAnotherPoint {
case let (x, y) where x == y:
print("(\(x), \(y)) is on the line x == y")
case let (x, y) where x == -y:
print("(\(x), \(y)) is on the line x == -y")
case let (x, y):
print("(\(x), \(y)) is just some arbitrary point")
}
// Prints "(1, -1) is on the line x == -y"
10. Compound Cases
- Switch문에서 알파벳을 입력해서 모음과 자음을 구분하는 예시입니다.
<swift />
let someCharacter: Character = "e"
switch someCharacter {
case "a", "e", "i", "o", "u":
print("\(someCharacter) is a vowel")
case "b", "c", "d", "f", "g", "h", "j", "k", "l", "m",
"n", "p", "q", "r", "s", "t", "v", "w", "x", "y", "z":
print("\(someCharacter) is a consonant")
default:
print("\(someCharacter) isn't a vowel or a consonant")
}
// Prints "e is a vowel"
- Switch문에서 Value Bindings를 사용한 예시입니다.
<swift />
let stillAnotherPoint = (9, 0)
switch stillAnotherPoint {
case (let distance, 0), (0, let distance):
print("On an axis, \(distance) from the origin")
default:
print("Not on an axis")
}
// Prints "On an axis, 9 from the origin"
11. Control Transfer Statements
- Switch에서는 아래와 같은 5가지의 제어 전달문을 가지고 있습니다.
- continue
- break
- fallthrough
- return
- throw
12. Continue
- Switch에서 continue는 조건을 만족할 시 현재의 반복이 즉시 종료되고 다음 반복의 시작으로 점프하게 되는 것을 말하며 아래의 예시와 같습니다.
<swift />
let puzzleInput = "great minds think alike"
var puzzleOutput = ""
let charactersToRemove: [Character] = ["a", "e", "i", "o", "u", " "]
for character in puzzleInput {
if charactersToRemove.contains(character) {
continue
}
puzzleOutput.append(character)
}
print(puzzleOutput)
// Prints "grtmndsthnklk"
13. Break
- Switch에서 baeak는 전제 제어 흐름 명령문의 실행을 즉시 종료하는 것을 말합니다. 아래의 예시와 같습니다.
<swift />
let numberSymbol: Character = "三" // Chinese symbol for the number 3
var possibleIntegerValue: Int?
switch numberSymbol {
case "1", "١", "一", "๑":
possibleIntegerValue = 1
case "2", "٢", "二", "๒":
possibleIntegerValue = 2
case "3", "٣", "三", "๓":
possibleIntegerValue = 3
case "4", "٤", "四", "๔":
possibleIntegerValue = 4
default:
break
}
if let integerValue = possibleIntegerValue {
print("The integer value of \(numberSymbol) is \(integerValue).")
} else {
print("An integer value couldn't be found for \(numberSymbol).")
}
// Prints "The integer value of 三 is 3.
14. fallthrough
- Switch에서 fallthrough문을 사용 시 다음 case문의 일치 여부와 상관없이 실행됩니다. 아래의 예시와 같습니다.
<swift />
let integerToDescribe = 5
var description = "The number \(integerToDescribe) is"
switch integerToDescribe {
case 2, 3, 5, 7, 11, 13, 17, 19:
description += " a prime number, and also"
fallthrough
default:
description += " an integer."
}
print(description)
// Prints "The number 5 is a prime number, and also an integer."
15. Labeled Statements
- 기본적으로 아래의 형태와 같습니다.
<swift />
label name: while condition {
statements
}
- 이를 이용하여 아까와 같이 뱀과 사다리 게임을 해결한 예시입니다.
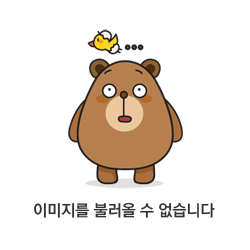
<swift />
let finalSquare = 25
var board = [Int](repeating: 0, count: finalSquare + 1)
board[03] = +08; board[06] = +11; board[09] = +09; board[10] = +02
board[14] = -10; board[19] = -11; board[22] = -02; board[24] = -08
var square = 0
var diceRoll = 0
gameLoop: while square != finalSquare {
diceRoll += 1
if diceRoll == 7 { diceRoll = 1 }
switch square + diceRoll {
case finalSquare:
// diceRoll will move us to the final square, so the game is over
break gameLoop
case let newSquare where newSquare > finalSquare:
// diceRoll will move us beyond the final square, so roll again
continue gameLoop
default:
// this is a valid move, so find out its effect
square += diceRoll
square += board[square]
}
}
print("Game over!")
16. Early Exit
- 기본적으로 아래의 예시와 같습니다.
<swift />
func greet(person: [String: String]) {
guard let name = person["name"] else {
return
}
print("Hello \(name)!")
guard let location = person["location"] else {
print("I hope the weather is nice near you.")
return
}
print("I hope the weather is nice in \(location).")
}
greet(person: ["name": "John"])
// Prints "Hello John!"
// Prints "I hope the weather is nice near you."
greet(person: ["name": "Jane", "location": "Cupertino"])
// Prints "Hello Jane!"
// Prints "I hope the weather is nice in Cupertino."
17. Checking API Availability
- Swift는 API 가용성을 확인하는 기능이 내장되어 있어 특정 배포 대상에서 사용할 수 없는 API를 실수로 사용하지 않도록 할 수 있습니다. 아래는 10 이상이 버전만 작동하는 예시입니다.
<swift />
if #available(iOS 10, macOS 10.12, *) {
// Use iOS 10 APIs on iOS, and use macOS 10.12 APIs on macOS
} else {
// Fall back to earlier iOS and macOS APIs
}
- 기본적인 API 가용성 확인 형태입니다.
<swift />
if #available(platform name version, ..., *) {
statements to execute if the APIs are available
} else {
fallback statements to execute if the APIs are unavailable
}
내용은 https://docs.swift.org/swift-book/LanguageGuide/TheBasics.html를 보면서 작성하였고 원문으로 작성된 내용을 옮기다 보니 이상한 부분이 있을 수 있습니다. 자세한 내용은 위의 링크를 확인해주시기 바랍니다.
'컴퓨터 언어 > Swift' 카테고리의 다른 글
(Swift) 6. Closures (클로저) (0) | 2021.12.09 |
---|---|
(Swift) 5. Functions (함수) (0) | 2021.12.07 |
(Swift) 3. Collection Types (컬렉션 타입) (0) | 2021.12.06 |
(Swift) 2. Strings and Characters (문자열과 문자) (0) | 2021.12.05 |
(Swift) 1. Basic Operators (기본 연산자) (0) | 2021.12.05 |